Lisa is a desktop computer developed by Apple, released on January 19, 1983.It is one of the first personal computers to present a graphical user interface (GUI) in a machine aimed at individual business users. Development of the Lisa began in 1978, and it underwent many changes during the development period before shipping at US$9,995 with a five-megabyte hard drive.
- Recursion Plaza Mac Os X
- Recursion Plaza Mac Os Download
- Recursion Plaza Mac Os Catalina
- Recursion Plaza Mac Os 11
PEP: | 651 |
---|---|
Title: | Robust Stack Overflow Handling |
Author: | Mark Shannon |
Status: | Rejected |
Type: | Standards Track |
Created: | 18-Jan-2021 |
Post-History: | 19-Jan-2021 |
- I create bespoke logos, flyers, infographics and signs for individuals, clubs and small businesses. I also provide 3D printing design and manufacturing services.
- This is my workable one. On mac OS X 10.10.4. Grep -e 'this' -rl. xargs sed -i ' 's/this/that/g' The above ones use find will change the files that do not contain the search text (add a new line at the file end), which is verbose.
- Does not seem to be an option (using Mac OS X 10.10.3). – pdeli Apr 15 '15 at 11:04. Add a comment 2 Answers Active Oldest Votes. In order to successfully copy the files in the first place, you need at least have read access to the files at the original location. To make sure that you can.
- 2.1 How to get R.app. R.APP is part of the binary distribution of R for Mac OS X available from CRAN.That distribution consists of one package containing the R framework and R.APP. Development versions of R.APP are made available on daily basis in the form of a disk image containing the R.APP itself. See the Mac OS X pages on CRAN for detail how to obtain such snapshots (currently at http.
Contents
- Specification
- C-API
- Implementation
This PEP has been rejected by the Python Steering Council.
This PEP proposes that Python should treat machine stack overflow differently from runaway recursion.
This would allow programs to set the maximum recursion depth to fit their needsand provide additional safety guarantees.
If this PEP is accepted, then the following program will run safely to completion:
and the following program will raise a StackOverflow, without causing a VM crash:
CPython uses a single recursion depth counter to prevent both runaway recursion and C stack overflow.However, runaway recursion and machine stack overflow are two different things.Allowing machine stack overflow is a potential security vulnerability, but limiting recursion depth can prevent theuse of some algorithms in Python.
Currently, if a program needs to deeply recurse it must manage the maximum recursion depth allowed,hopefully managing to set it in the region between the minimum needed to run correctly and the maximum that is safeto avoid a memory protection error.
By separating the checks for C stack overflow from checks for recursion depth,pure Python programs can run safely, using whatever level of recursion they require.
CPython currently relies on a single limit to guard against potentially dangerous stack overflowin the virtual machine and to guard against run away recursion in the Python program.
This is a consequence of the implementation which couples the C and Python call stacks.By breaking this coupling, we can improve both the usability of CPython and its safety.
The recursion limit exists to protect against runaway recursion, the integrity of the virtual machine should not depend on it.Similarly, recursion should not be limited by implementation details.
Two new exception classes will be added, StackOverflow and RecursionOverflow, both of which will besub-classes of RecursionError
StackOverflow exception
A StackOverflow exception will be raised whenever the interpreter or builtin module codedetermines that the C stack is at or nearing a limit of safety.StackOverflow is a sub-class of RecursionError,so any code that handles RecursionError will handle StackOverflow
RecursionOverflow exception
A RecursionOverflow exception will be raised when a call to a Python functioncauses the recursion limit to be exceeded.This is a slight change from current behavior which raises a RecursionError.RecursionOverflow is a sub-class of RecursionError,so any code that handles RecursionError will continue to work as before.
Decoupling the Python stack from the C stack
In order to provide the above guarantees and ensure that any program that worked previouslycontinues to do so, the Python and C stack will need to be separated.That is, calls to Python functions from Python functions, should not consume space on the C stack.Calls to and from builtin functions will continue to consume space on the C stack.
The size of the C stack will be implementation defined, and may vary from machine to machine.It may even differ between threads. However, there is an expectation that any code that could runwith the recursion limit set to the previous default value, will continue to run.
Many operations in Python perform some sort of call at the C level.Most of these will continue to consume C stack, and will result in aStackOverflow exception if uncontrolled recursion occurs.
Other Implementations
Other implementations are required to fail safely regardless of what value the recursion limit is set to.
If the implementation couples the Python stack to the underlying VM or hardware stack,then it should raise a RecursionOverflow exception when the recursion limit is exceeded,but the underlying stack does not overflow.If the underlying stack overflows, or is near to overflow,then a StackOverflow exception should be raised.
C-API
A new function, Py_CheckStackDepth() will be added, and the behavior of Py_EnterRecursiveCall() will be modified slightly.
Py_CheckStackDepth()
int Py_CheckStackDepth(const char *where)will return 0 if there is no immediate danger of C stack overflow.It will return -1 and set an exception, if the C stack is near to overflowing.The where parameter is used in the exception message, in the same fashionas the where parameter of Py_EnterRecursiveCall().
Py_EnterRecursiveCall()
Py_EnterRecursiveCall() will be modified to call Py_CheckStackDepth() before performing its current function.
PyLeaveRecursiveCall()
Py_LeaveRecursiveCall() will remain unchanged.
This feature is fully backwards compatibile at the Python level.Some low-level tools, such as machine-code debuggers, will need to be modified.For example, the gdb scripts for Python will need to be aware that there may be more than one Python frameper C frame.
C code that uses the Py_EnterRecursiveCall(), PyLeaveRecursiveCall() pair offunctions will continue to work correctly. In addition, Py_EnterRecursiveCall()may raise a StackOverflow exception.
New code should use the Py_CheckStackDepth() function, unless the code wants tocount as a Python function call with regard to the recursion limit.
We recommend that 'python-like' code, such as Cython-generated functions,use Py_EnterRecursiveCall(), but other code use Py_CheckStackDepth().
It will no longer be possible to crash the CPython virtual machine through recursion.
It is unlikely that the performance impact will be significant.
The additional logic required will probably have a very small negative impact on performance.The improved locality of reference from reduced C stack use should have some small positive impact.
It is hard to predict whether the overall effect will be positive or negative,but it is quite likely that the net effect will be too small to be measured.
Monitoring C stack consumption
Gauging whether a C stack overflow is imminent is difficult. So we need to be conservative.We need to determine a safe bounds for the stack, which is not something possible in portable C code.
For major platforms, the platform specific API will be used to provide an accurate stack bounds.However, for minor platforms some amount of guessing may be required.While this might sound bad, it is no worse than the current situation, where we guess that thesize of the C stack is at least 1000 times the stack space required for the chain of calls from_PyEval_EvalFrameDefault to _PyEval_EvalFrameDefault.
This means that in some cases the amount of recursion possible may be reduced.In general, however, the amount of recursion possible should be increased, as many calls will use no C stack.
Our general approach to determining a limit for the C stack is to get an address within the current C frame,as early as possible in the call chain. The limit can then be guessed by adding some constant to that.
Making Python-to-Python calls without consuming the C stack
Calls in the interpreter are handled by the CALL_FUNCTION,CALL_FUNCTION_KW, CALL_FUNCTION_EX and CALL_METHOD instructions.The code for those instructions will be modified so that whena Python function or method is called, instead of making a call in C,the interpreter will setup the callee's frame and continue interpretation as normal.
The RETURN_VALUE instruction will perform the reverse operation,except when the current frame is the entry frame of the interpreterwhen it will return as normal.
None, as yet.
This document is placed in the public domain or under theCC0-1.0-Universal license, whichever is more permissive.
Source: https://github.com/python/peps/blob/master/pep-0651.rstCourse Information
Overview
CS 2112/ENGRD 2112 is an honors version ofCS 2110/ENGRD 2110. Credit is given for only oneof 2110 and 2112. Transfer between 2110 and 2112 (in either direction) isencouraged during the first three weeks. We cover intermediate software design andintroduce some key computer science ideas. The topics are similar to those in2110 but are covered in greater depth with more challenging assignments.Topics include object-oriented programming, program structure andorganization, program reasoning using specifications and invariants, recursion,design patterns, concurrent programming, graphical user interfaces, datastructures, sorting and graph algorithms, asymptotic complexity, and simplealgorithm analysis. Java is the principal programming language.
Time & Place
Lecture:
- Tuesdays and Thursdays, 10:10-11am, Gates G01(ground floor, below the main entrance).
Labs:
- Mondays 12:20-1:10pm, Hollister 206 or
- Mondays 7:30-8:20pm, Upson 206 or
- Wednesdays 7:30-8:20pm, Upson 206
Discussions:
- Tuesdays 12:20-1:10pm, Hollister 206 or
- Wednesdays 1:25-2:15pm, Hollister 206 or
- Wednesdays 3:35-4:25pm, Hollister 372
You are expected to attend all lectures, one lab, and one discussion each week.
Course Staff
Office Hours Weekly Chart
Day | Name | Time | Location |
---|---|---|---|
Monday | Richard | 11am-12pm | Gates G17 |
Olivia | 1-2pm | Gates G15 | |
Sixian | 4-5pm | Gates G15 | |
Gautam | 6-7pm | Gates G11 | |
Tuesday | Dexter | 11:15am-12pm | Gates 406 |
Travis | 1:30-2:30pm | Gates G17 | |
Jane | 4:30-5:30pm | Gates G15 | |
Wilson | 7:30-8:30pm | Gates G11 | |
Wednesday | Kenneth | 2:30-3:30pm | Gates G19 |
Talha | 4:30-5:30pm | Gates G19 | |
Yiteng | 6-7pm | Gates G19 | |
Thursday | Kevin | 9-10am | Gates G15 |
Dexter | 11:15am-12pm | Gates 310 | |
Jacob | 1-2pm | Gates G15 | |
Shiyu | 6-7pm | Gates G19 | |
Friday | Albert | 11am-12pm | Gates G19 |
Alan | 12-1pm | Gates G13 | |
John | 1-2pm | Gates G15 | |
Lucas | 3:30-4:30pm | Gates G15 | |
Sunday | Kenneth | 3:30-4:30pm | Tatkon 3343 |
Newton | 8-9pm | Gates G15 |
Prerequisites
Very good performance in CS 1110 or CS 1130 or an equivalent course,or permission of the instructor. If you are unsure whether CS 2110 or CS2112 is the right course for you, please talk to the instructor of eithercourse. Both courses cover similar material and satisfy the same requirements,but CS 2112 covers material in more depth and has more challenging assignments.It is aimed at computer science majors.
Texts
You are required to read the course notes posted on the web site. These will often contain moredetail than what was presented in lecture.
There is a recommended textbook, which is also the textbook for CS 2110.It is useful especially for examples of how to implement various data structures.
- • Data Structures and Abstractions with Java, 4th edition, Frank M. Carrano and Timothy Henry, Pearson Education, 2014. ISBN-10: 0133744051, ISBN-13: 9780133744057.
See also the companion website for additional material.
Other Sources
- • Data Structures and Problem Solving Using Java, 3rd edition, Mark Allen Weiss, Addison Wesley, 2006. ISBN 0-321-32213-4. See also Weiss's website for additional material. A required text from previous years.
- • Program development in Java: Abstraction, Specification, and Object-Oriented Design, B. Liskov and J. Guttag, Addison-Wesley, 2000. ISBN 0-201-65768-6. An excellent source of material on designing and specifying abstractions.
- • Java Precisely, 2nd edition, P. Sestoft, MIT Press, 2005. To access the entire book for free, login via the Cornell Engineering Library.
- • Design Patterns, Erich Gamma, Richard Helm, Ralph Johnson, and John M. Vlissides, Addison Wesley, 1994. ISBN 0-201-63361-2. An extremely influential book on software engineering. According to Wikipedia, as of April 2007, the book was in its 36th printing and has sold over 500,000 copies in English and 13 other languages.
- • Java in a Nutshell, 5th edition, David Flanagan, O'Reilly, 2005. ISBN 0-596-00773-6.
These titles are on reserve in the Engineering library, Carpenter Hall.
The CS 2110 website has many online learning aids and tutorial videos, including introductory videos on Java and Eclipse.
Piazza
We will be using Piazza as an online discussion forum. You are encouraged to post any questions you might have about the course material. The course staff monitor Piazza fairly closely and you will usually get a quick response. If you know the answer to a question, you are encouraged to post it, but please avoid giving away any hints on the homework or posting any part of a solution—this is considered a violation of academic integrity.
By default, your posts are visible to the course staff and other students, and you should prefer this mode so that others can benefit from your question and the answer. However, you can post privately so that only the course staff can see your question, and you should do so if your post might reveal information about a solution to a homework problem. You can also post anonymously if you wish. If you post privately, we reserve the right to make your question public if we think the class will benefit.
Piazza is the most effective way to communicate with the staff and is the preferred mode of interaction. Please reserve email for urgent or confidential matters. Free-ranging technical discussions are especially encouraged. Broadcast messages from the course staff to students will be sent using Piazza and all course announcements will be posted there, so check in often.
CMS
We will be using the course management system CMS for managing assignments, exams, and grades. Everyone who preregistered for the course should be entered, but if you did not preregister, you are probably missing. Please login here and check whether you exist. There will be a list of courses you are registered for, and CS 2112 should be one of them. If not, please send your full name and Cornell netId to the Administrative Assistant or so that you can be registered.
You can check your grades, submit homework, and request regrades in CMS. Please check your grades regularly to make sure we are recording things properly. The system also provides some grading statistics. There is a help page with instructions.
Please do not repost course materials released on CMS publicly. These materials are intellectual property and are meant for participants in the course. They are not free to the public.
Announcements and Handouts
Announcements will be posted to Piazza. Homework and exam solutions will be available in CMS. Check frequently for new postings.
Assignments
There will be seven assignments, mostly involving programming but also some writtenproblems. These assignments will make up 40% of your score. The first three assignments will bedone alone. The last four assignments are parts of a single project, which will be done with a partner.
Unless otherwise specified, assignments may be turned in late with adeduction of 10% per day up to a maximum of four days.
Avoid the bomb ! mac os. Extensions are granted in case of illness or other acceptable excuse.
Exams
There will be one evening prelim and a final exam. Please check the schedule page for times and locations. The prelim will be 20% of your score and the final will be 35%.
Regrades
Graded homework will be available on CMS. Graded exams will be available in the handback room, 216 Gates.
Homework regrade requests can be submitted electronically in CMS. Exam regrades should be handwritten and submitted to the course staff. Please include a description of the grading error with your regrade request.
Academic Integrity
The utmost level of academic integrity is expected of all students. Under no circumstances may you submit work done with or by someone else under your own name or share detailed proofs or code with anyone else except your partner. However, questions regarding general techniques or the requirements of the assignment are permissible.
You must cite all sources, including Internet sources. You must acknowledge by name anyone whom you consulted.You may not give nor receive assistance from anyone else during an exam.You may not give any hints or post any material that might be part of a solution publicly on Piazza. If your question necessarily includes such material, post privately.
If you are unsure about what is permissible and what is not, please ask.
Academic Integrity Resources:
- • Cornell University Code of Academic Integrity
- • Computer Science Department Code of Academic Integrity
- • Explanation of AI Proceedings
Special Needs
We will provide appropriate accommodations for students with special needs and/or disabilities. Requests for accommodations are to be made during the first three weeks of the semester and must be accompanied by official documentation. Please register with Student Disability Services in 110 Ho Plaza (Cornell Health Building), Level 5 to document your eligibility.
Placeholder for schedule
Resources
Course notes
Course notes for individual lectures and recitations can be found on thecourse schedule. The notes arealso available as a single printable document.
Java
Java is one of the most widely used object-oriented programming languages, and programming skill in the Java language is in high demand. Nevertheless, this is not a course about Java. Java is simply a good vehicle for explaining many of theideas on data structures, algorithms, and software engineering that will be covered in the course. Most of the ideas you will be exposed to in this course,and the skills you will develop, will transfer to other programming languages.
Online Resources
• CS 2110 has a comprehensive collection of online modules introducing Java and Eclipse, along with other resources.
• The Java API contains documentation for the extensive Java class library.
• The Java Language Specification is helpful if youwant to really understand how Java works.
• Oracle has an official Java Tutorial.
Brushing Up
Review the introductory chapters in the textbook and the Java reference books listed on the course info page. For students with C++ experience, check out this Wikipedia page comparing C++ with Java.
Java version
We will be using the Java 8 Standard Edition (Java SE 8) platform, whichconsists of the Java Development Kit (JDK) and the Java Runtime Environment(JRE). If you are using Java 7 or earlier, please upgrade.
To find out which version of the JRE you arerunning, open a command window (in Windows, Start > Run.. and type cmd
,and in Mac OS, Applications > Utilities > Terminal)and type java -version
at the command prompt:
This says I have version 8 installed (8 and 1.8 are synonymous).
If you are on a PC running Windows and have never installed a versionof the Java Development Kit (JDK) on your machine, you probably don't have it.If you are on a Mac, you probably do. To find out, type javac -version
:
If you get an error message or the version is earlier than 1.8, you must (re)install the JDK.
Installing the JDK
The JDK is already installed in CIT and ACCEL labs. However, installing it ownyour own machine will greatly facilitate your work.
To download the JDK, visit Oracle's Java web site and download and install the appropriate version of the JDK 8 for your platform.
Compiling and Running from the Command Line
Compiling: Say your main class is MyProgram
and it iscontained in the source file MyProgram.java
.If it is not in a package, navigateto the folder containing MyProgram.java
and type javac MyProgram.java
. If it is in a package (say myPackage
), thesource should be in a folder called myPackage
. Navigateto the folder containing myPackage
and type javac myPackage/MyProgram.java
.
Samurai anki mac os. Running: From the same folder you compiled from, typejava MyProgram <program arguments>
if it is notin a package, and java myPackage.MyProgram <program arguments>
if it is.
Specifying a Classpath: Sometimes you may need to inform Java where to find auxiliaryclasses. You can do this with the -cp
optionto the java
command.Supply a sequence of folders telling Java where to lookfor classes, separated by :
(Mac) or;
(Windows).
Local Software
Some Java libraries have been developed for use in CS 2110 and CS 2112 assignments.Feel free to use them.
EasyIO
: Support for easy console input and output, and for scanninginput from a file or string (like java.util.Scanner
, but more powerful).[ doc | jar | source ]
cs2110
: Various types of queues, including a priority queue supporting in situ adjustment of priorities;a DelimitedStringBuilder similar to one found in OCaml; a module that runs an external script that takes astring on stdin and writes a string to stdout; and a module for easy access to a text resource file.[ doc | jar | source ]
IDEs
IDE stands for integrated development environment.The use of an IDE is the best way to develop Java programs.IDEs provide many valuable aids such as syntax checking,debugging, and refactoring that can save you a lot of effort.
There are many good IDEs. We recommend Eclipse, but youmay use any one that you like, or none at all.Eclipse is installed in all the labs, along with some others.Early recitation sections will get you started with Eclipseif you are not familiar with an IDE.
Here are some links:
For consistency, the course staff use Eclipse with a common Java style. Youshould follow this style guide. You can download the Eclipse style templatehere.
Programming
There are many valuable resources that can help you take your programmingskills to the next level. Here are a few links:
General
Software Development Methodologies
Debugging
Just for Fun
Computer Labs
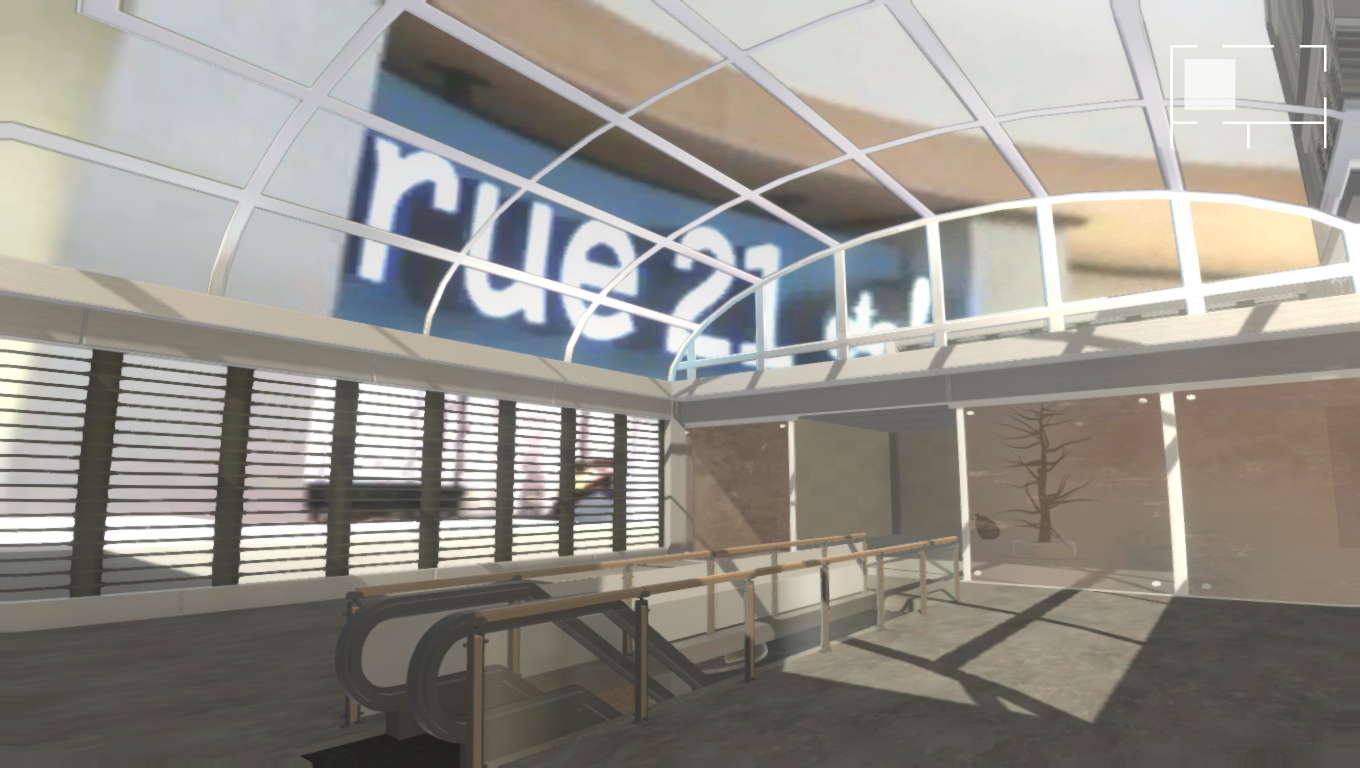
CIT Labs
Cornell Information Technologies (CIT) runs several computer labs across campus for all members of the Cornell community. The JDK and Eclipse are installed on these machines. Check here for locations and times of operation.
ACCEL Lab
You can also find the course software in the Academic Computing Center (ACCEL), located in the Engineering Library in Carpenter Hall. Any CS student may register for an account.
Support Services
Recursion Plaza Mac Os X
Academic Excellence Workshops
The Academic Excellence Workshops (AEW) offer an opportunity for students to gain additional experience with course concepts in a cooperative learning environment. Research has shown that cooperative and collaborative methods promote higher grades, greater persistence, and deeper comprehension. The material presented in the workshop is at or above the level of the regular course. We do not require joining the AEW program, but do encourage students to join if they are seeking an exciting and fun way to learn. The AEW carries one S/U credit based on participation and attendance. The time commitment is two hours per week in the lab. No homework will be given. This is a wonderful opportunity for students to seek extra help on course topics in a small group setting.
Your fellow undergraduate students, who are familiar with the course material, teach the sessions with material that they prepare. The course staff provide guidance and support but do not actually teach the AEW course content or any session. A representative from the AEW program will be speaking about the program and registration procedures in lecture.
One of your TAs will be designated as the AEW liaison for CS 2112. Watch theannouncements to find out who.
See the AEW webpage for further information.
Other Support Services
Recursion Plaza Mac Os Download
PROGRAM | DESCRIPTION |
---|---|
Registrar's Office | General services for the Cornell community. |
Engineering College | Support services for Engineering students. |
Arts College | Support services for Arts students. |
CIT | Services offered by Cornell Information Technologies (CIT), including computer training. |
Cornell Health | The Cornell University Health Service Center. For all health related concerns and counseling services. |
Learning Styles | Not everyone learns the same way. If you are curious about how you learn, check out this collection. |
Assignments
All source code will be available on CMS.
Assignment 1 | Introduction to Java and object-oriented languages | |
Assignment 2 | Ciphers and EncryptionLast update 9/10 6:36pm | Design document |
Assignment 3 | Data Structures and Text Editing | |
Assignment 4 | Parsing and Fault InjectionLast update 10/13 10:27am | Design document |
Assignment 5 | Interpretation and SimulationLast update 11/11 3:55pm | Design document |
Assignment 6 | Graphical User Interface DesignLast update 11/6 1:15pm | Design document |
Assignment 7 | Distributed and Concurrent Programming | Design document |
Recursion Plaza Mac Os Catalina
Other resources for programming assignments:
Recursion Plaza Mac Os 11
- Group project specification:Simulating Evolving Artificial Life